Using Enums in Golang: Best Practices and Examples
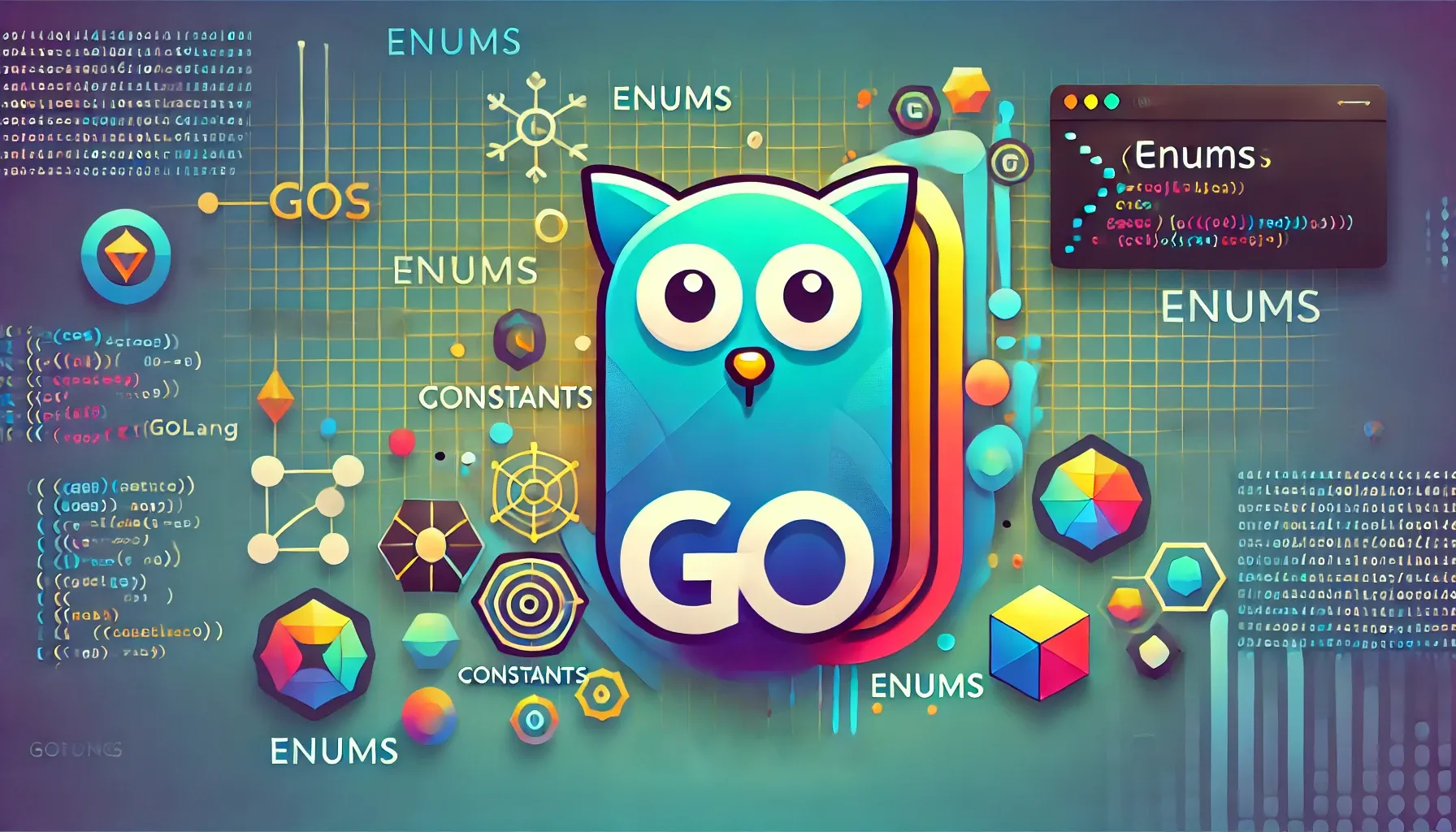
Golang (Go) is known for its simplicity and performance-oriented design. However, Go does not have a built-in enum
type. Instead, developers can create enum-like structures using constants and custom types. In this article, we'll explore how to implement enums in Go, how to use them effectively, and some best practices for working with them.
What is an Enum?
An enum (short for enumeration) is a data type that represents a set of predefined constants. For example, you might use an enum to represent the days of the week:
- Monday
- Tuesday
- Wednesday ...
In Go, enums can be implemented using const
and iota
.
How to Create Enums in Go
Enums in Go are typically created using const
and iota
. Here's an example:
package main
import "fmt"
// Define a custom type for the enum
type Day int
const (
Monday Day = iota // 0
Tuesday // 1
Wednesday // 2
Thursday // 3
Friday // 4
Saturday // 5
Sunday // 6
)
// Add a String method for better readability
func (d Day) String() string {
switch d {
case Monday:
return "Monday"
case Tuesday:
return "Tuesday"
case Wednesday:
return "Wednesday"
case Thursday:
return "Thursday"
case Friday:
return "Friday"
case Saturday:
return "Saturday"
case Sunday:
return "Sunday"
default:
return "Unknown"
}
}
func main() {
var today Day = Friday
fmt.Println("Today is:", today)
}
Explanation
- We defined a custom type called
Day
. - Using
iota
, we created sequential constants starting from 0. - A
String()
method was added to provide human-readable names for each enum value.
Best Practices for Using Enums
1. Ensure Type Safety
Using a custom type instead of int
ensures type safety and prevents invalid values from being assigned.
func IsWeekend(day Day) bool {
return day == Saturday || day == Sunday
}
2. Iterate Over Enum Values
To iterate over all possible enum values, you can use a helper function:
func GetAllDays() []Day {
return []Day{Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday}
}
func main() {
for _, day := range GetAllDays() {
fmt.Println(day)
}
}
3. Add JSON Support
If you need to use enum values with JSON, implement the MarshalJSON
and UnmarshalJSON
methods.
import "encoding/json"
func (d Day) MarshalJSON() ([]byte, error) {
return json.Marshal(d.String())
}
func (d *Day) UnmarshalJSON(data []byte) error {
var dayStr string
if err := json.Unmarshal(data, &dayStr); err != nil {
return err
}
switch dayStr {
case "Monday":
*d = Monday
case "Tuesday":
*d = Tuesday
// Handle other cases...
default:
return fmt.Errorf("invalid day: %s", dayStr)
}
return nil
}
Conclusion
While Go does not have a built-in enum
type, you can create powerful and flexible enum-like structures using const
, iota
, and custom types. These structures improve type safety, readability, and extensibility in your code.
If you have experience using enums in your Go projects, feel free to share your insights and best practices in the comments!